Matthias_H
Member
- Joined
- May 21, 2009
- Messages
- 194
Dear all,
Quite a while ago I suggested the creation of a guitar simulation for the Pandora. The idea is to have the photo of guitar strings as GUI, and the user could use the stylus (or their fingers) to pluck or strum the strings. The actual pitch per string (fingering) could be controlled through key combinations, preprogrammed song structures, etc. Once the basic functionality is up and running, we can think about dedicated modes for different musical styles (Folk, Jazz, Campfire, Flamenco, Latin strummed, Latin plucked, ...), a network competition mode, guitar lessons, effects, more guitar models, etc.
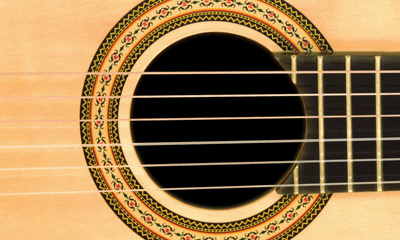
I am not a programmer (though most of the code I write somehow gets its job done) but there should be plenty of these around here. So if you're interested, feel free to join the discussion. On the other hand, I know quite a bit about physics and music and guitars. Given the skeleton of an audio engine / UI, I could contribute to the functionality to make everything behave like it should. Also, I have the equipment to record a multisample, (and I could even contribute a proper animation of vibrating guitar strings
).
Here's what I think are the two main TECHNICAL challenges that the engine would have to meet.
(1) Polyphonic low-latency playback of audio samples (at least 12 stereo voices). For decent quality, a pool of about 150 individual samples will be recorded (each a few seconds long), totalling at about 80MB of 44.1kHz/16bit/stereo sample data which, as I figure, would all fit into the RAM at once. The engine must be able to access all the data randomly in an instant, and play back samples at variable volume and pitch (50% - 150% of original speed). It would also be good to have an antialiasing filter for transposed playback.
Edit: Solved (see below
)
(2) GUI with touchpad handling. Essentially, the stylus position needs to be tracked at high rate. Whenever a string is crossed, the following function is fired.
Quite a few people seem to have gotten their Pandoras already (I haven't), so if there is any programmer out there who could hack together a basic engine, that'd be awesome. The very moment I receive my Pandora, I'm in
Cheers,
Matthias
Quite a while ago I suggested the creation of a guitar simulation for the Pandora. The idea is to have the photo of guitar strings as GUI, and the user could use the stylus (or their fingers) to pluck or strum the strings. The actual pitch per string (fingering) could be controlled through key combinations, preprogrammed song structures, etc. Once the basic functionality is up and running, we can think about dedicated modes for different musical styles (Folk, Jazz, Campfire, Flamenco, Latin strummed, Latin plucked, ...), a network competition mode, guitar lessons, effects, more guitar models, etc.
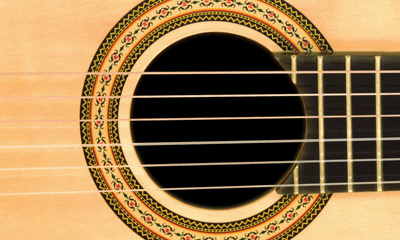
I am not a programmer (though most of the code I write somehow gets its job done) but there should be plenty of these around here. So if you're interested, feel free to join the discussion. On the other hand, I know quite a bit about physics and music and guitars. Given the skeleton of an audio engine / UI, I could contribute to the functionality to make everything behave like it should. Also, I have the equipment to record a multisample, (and I could even contribute a proper animation of vibrating guitar strings
Here's what I think are the two main TECHNICAL challenges that the engine would have to meet.
(1) Polyphonic low-latency playback of audio samples (at least 12 stereo voices). For decent quality, a pool of about 150 individual samples will be recorded (each a few seconds long), totalling at about 80MB of 44.1kHz/16bit/stereo sample data which, as I figure, would all fit into the RAM at once. The engine must be able to access all the data randomly in an instant, and play back samples at variable volume and pitch (50% - 150% of original speed). It would also be good to have an antialiasing filter for transposed playback.
Edit: Solved (see below
Code:
// fire a sample
void playSample(
int voice, // which playback channel?
short *data, int length, // address and size of the audio sample
float volume, float pitch, int offset = 0 // playback parameters
);
// change the pitch during playback
void changePitch(
int voice,
float pitch
);
// ramp down the volume of a sample
void dampenSample(
int voice,
float decay
);
(2) GUI with touchpad handling. Essentially, the stylus position needs to be tracked at high rate. Whenever a string is crossed, the following function is fired.
Code:
void pluckString(
int number, // which string was plucked?
float stylusVelocity, // something along the lines of "multiples of screen height per second"
float stringPosition // bridge: 0.f; end of fretboard: 1.f
) {
int velocity = stylusVelocity * SCALE_VELOCITY * NUMSAMPLES_VELOCITY;
int pitch = getStringPitch(number); // manage the fingering somehow
int note = getStringNote(number); // ...
int stringPos = stringPosition * NUMSAMPLES_STRINGPOS;
float volume = getVolume(stylusVelocity, stringPosition); // some scaling function
int sampleIndex = getSampleIndex(number, velocity, note, stringPos);
playSample(number, samples[sampleIndex], sampleLengths[sampleIndex], volume, pitch, 0);
}
Quite a few people seem to have gotten their Pandoras already (I haven't), so if there is any programmer out there who could hack together a basic engine, that'd be awesome. The very moment I receive my Pandora, I'm in
Cheers,
Matthias
Last edited by a moderator: