sebt3
homebrew player (P. & C.)
Hi there,
I want my next game to play the same on the caanoo and pandora. As you (might) know, the caanoo have a 320x240 res.
So I'm planning for a game that can play in that resolution up to 400x240 (I'll find something to do with these extra pixels) and plan on scalling up for the pandora.
So for testing I'm building a test tilemap (randomly generated) and scale use the projection matrix :
EGE_viewport.cur to EGE_viewport.br is always 400x240 while the viewport is 800x480 obviously.
Here the result :
that's blurry, so let's scale the texture 2x before hand :
And everything is like the plan.... beside these nasty vertical lines.
when not scaled, these lines arent there, I even tryed to blit 22x22 square every 20px to be sure to overlap, and these lines are still there.
So guys, what am I missing ? Is there a way to remove these ?
I want my next game to play the same on the caanoo and pandora. As you (might) know, the caanoo have a 320x240 res.
So I'm planning for a game that can play in that resolution up to 400x240 (I'll find something to do with these extra pixels) and plan on scalling up for the pandora.
So for testing I'm building a test tilemap (randomly generated) and scale use the projection matrix :
Code:
glMatrixMode(GL_PROJECTION);
glLoadIdentity();
glOrtho(EGE_viewport.cur[0], EGE_viewport.br[0], EGE_viewport.br[1], EGE_viewport.cur[1], 1, -1);
EGE_viewport.cur to EGE_viewport.br is always 400x240 while the viewport is 800x480 obviously.
Here the result :
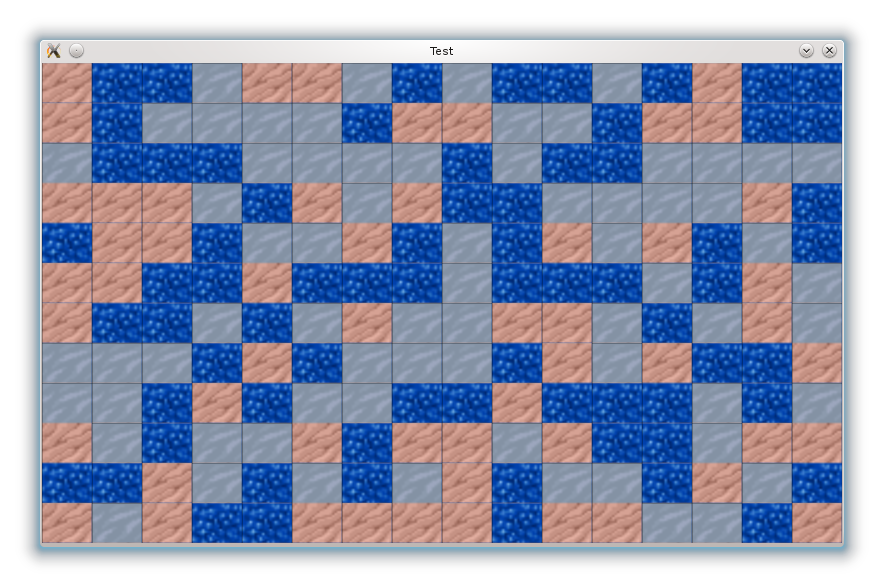
that's blurry, so let's scale the texture 2x before hand :
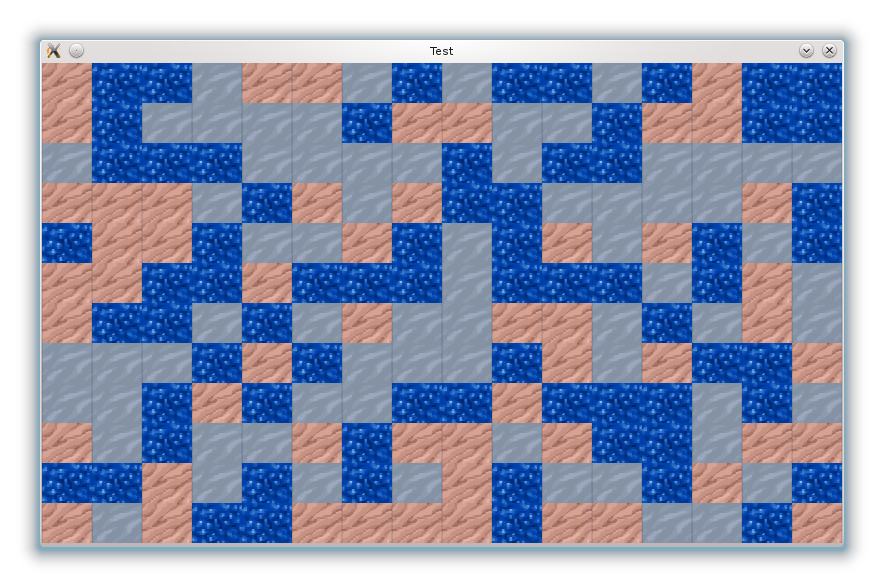
And everything is like the plan.... beside these nasty vertical lines.
when not scaled, these lines arent there, I even tryed to blit 22x22 square every 20px to be sure to overlap, and these lines are still there.
So guys, what am I missing ? Is there a way to remove these ?