RndmNumGenerator
Still Fresh
- Joined
- Sep 2, 2011
- Messages
- 4
I'd decided to pick up Python over the summer so I could begin working on app development for android phones. After getting the basics down, my first project was making a simple adventure roguelike game using the Pygame library, but I'm having problems with the pygame graphics I can't work out.
Basing my game off of tutorials and example code, I've got everything working so far except rendering the sprites(or sprite, more accurately. At this point I only have the player in the game). I can render him to the screen just fine, but the problem is when I move him - he gets rendered at his new location, but doesn't stop being rendered where he was previously. So when he moves, he leaves a trail of himself behind.
I think I could just re-render the entire background to cover the old sprites up, but that seems horribly inefficient. Is there a way to just re-render the parts that need it? Or just clean up old instances of the sprite? Before Python I was using ActionScript, where all you needed to do was move the sprite's x and y position and Flash would do all the rest... I guess Python needs something more.
I would appreciate any help you could give.
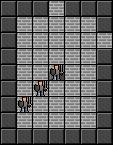
Basing my game off of tutorials and example code, I've got everything working so far except rendering the sprites(or sprite, more accurately. At this point I only have the player in the game). I can render him to the screen just fine, but the problem is when I move him - he gets rendered at his new location, but doesn't stop being rendered where he was previously. So when he moves, he leaves a trail of himself behind.
I think I could just re-render the entire background to cover the old sprites up, but that seems horribly inefficient. Is there a way to just re-render the parts that need it? Or just clean up old instances of the sprite? Before Python I was using ActionScript, where all you needed to do was move the sprite's x and y position and Flash would do all the rest... I guess Python needs something more.
I would appreciate any help you could give.
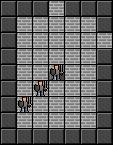